1.다음은 HTML 페이지와 브라우저의 출력결과이다.
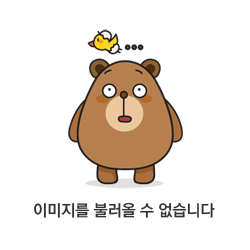
<!DOCTYPE html>
<html>
<head>
<title>라면을 끓이는 순서</title>
</head>
<body>
<h3>라면을 끓이는 순서</h3>
<hr>
<ol type="A">
<li>물을 끓인다.</li>
<li>라면과 스프를 넣는다.</li>
<li>파를 썰어 넣는다.</li>
<li>5분후 <strong>맛있게</strong> 먹는다.</li>
</ol>
</body>
</html>
(1)HTML페이지의 HTML DOM트리를 그려라.
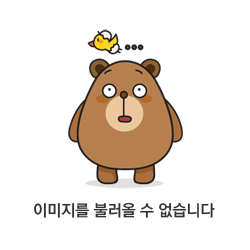
(2)</ol>dhk </body>태그 사이에 다음과 같이 자바스크립트 코드를 작서아여 모든 <li>태그를 찾아 글자 색을 green으로 출력하라.
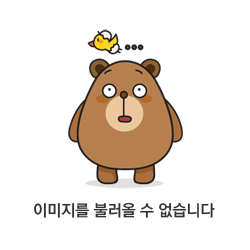
<!DOCTYPE html>
<html>
<head>
<title>라면을 끓이는 순서</title>
</head>
<body>
<h3>라면을 끓이는 순서</h3>
<hr>
<ol type="A">
<li>물을 끓인다.</li>
<li>라면과 스프를 넣는다.</li>
<li>파를 썰어 넣는다.</li>
<li>5분후 <strong>맛있게</strong> 먹는다.</li>
<script>
var liArray = document.getElementsByTagName("li");
for(var i=0 ; i<liArray.length ; i++){
var li = liArray[i];
li.style.color = "green";
}
</script>
</ol>
</body>
</html>
(3)리스트가 출력된 공간 아무 곳이나 클릭하면 리스트 전체의 배경색을 yellowgreen으로 바꾸는 자바스크립트 코드를 완성하라.
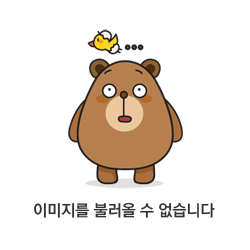
<!DOCTYPE html>
<html>
<head>
<title>라면을 끓이는 순서</title>
</head>
<script>
function change(obj){
obj.style.backgroundColor="yellowgreen";
}
</script>
<body>
<h3>라면을 끓이는 순서</h3>
<hr>
<ol type="A" onclick="change(this)">
<li>물을 끓인다.</li>
<li>라면과 스프를 넣는다.</li>
<li>파를 썰어 넣는다.</li>
<li>5분후 <strong>맛있게</strong> 먹는다.</li>
</ol>
</body>
</html>
<!DOCTYPE html>
<html>
<head>
<title>라면을 끓이는 순서</title>
</head>
<script>
</script>
<body>
<h3>라면을 끓이는 순서</h3>
<hr>
<ol type="A" onclick="this.style.backgroundColor='yellowgreen'">
<li>물을 끓인다.</li>
<li>라면과 스프를 넣는다.</li>
<li>파를 썰어 넣는다.</li>
<li>5분후 <strong>맛있게</strong> 먹는다.</li>
</ol>
</body>
</html>
2.다음은 HTML 페이지와 브라우저 출력결과이다.
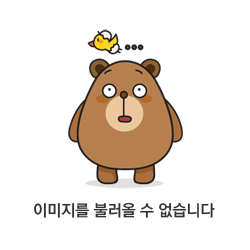
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>자바스크립트 연습</title>
</head>
<body>
<h3>자바스크립트란?
<button>border</button>
<button>p</button>
</h3>
<hr>
<p>자바스크립트는 웹 프로그래밍 언어로서 웹 페이지를 동적 변경시킬 수 있다.</p>
<p><span id="mySpan">C 언어</span>를 아는 사용자는 빠르게 학습할 수 있다.</p>
<p>이벤트 등 GUI 프로그래밍에 필요한 요소들이 있어 학습에 약간의 어려움이 있다.</p>
</body>
</html>
(1)HTML 페이지의 HTML DOM 트리를 그려라.
(2)"border"버튼을 클릭하면 다음과 같이 id="mySpan"인 <span>태그에 2픽셀의 violet 색 점선 테두리가 생기도록 자바스크립트 코드를 작성하라.
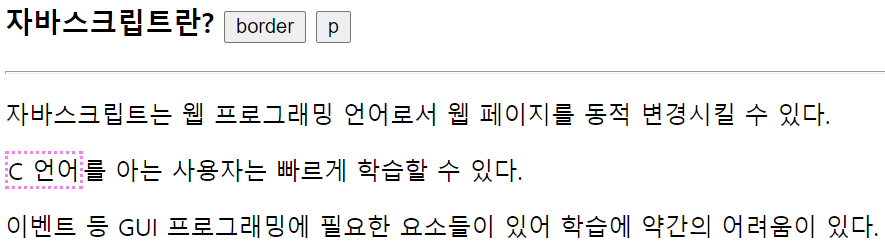
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>자바스크립트 연습</title>
<script>
function border(){
var obj = document.getElementById("mySpan");
obj.style.border="2px dotted violet";
}
</script>
</head>
<body>
<h3>자바스크립트란?
<button onclick="border()">border</button>
<button>p</button>
</h3>
<hr>
<p>자바스크립트는 웹 프로그래밍 언어로서 웹 페이지를 동적 변경시킬 수 있다.</p>
<p><span id="mySpan">C 언어</span>를 아는 사용자는 빠르게 학습할 수 있다.</p>
<p>이벤트 등 GUI 프로그래밍에 필요한 요소들이 있어 학습에 약간의 어려움이 있다.</p>
</body>
</html>
(3)"p"버튼을 클릭하면 <p>태그가 몇개 있는지 alert()를 통해 다음과 같이 출력되도록 자바스크립트 코드를 삽입하여 HTML 페이지를 수정하라.
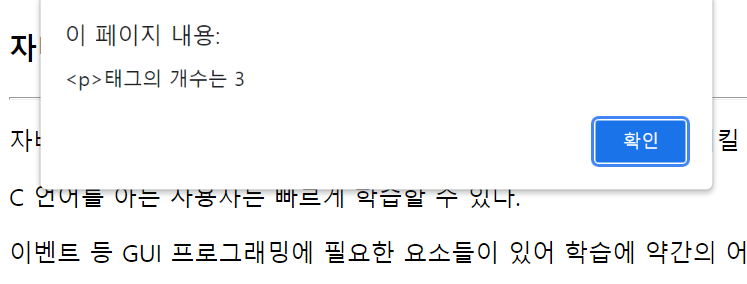
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>자바스크립트 연습</title>
<script>
function border(){
var obj = document.getElementById("mySpan");
obj.style.border="2px dotted violet";
}
function p(){
var pArray = document.getElementsByTagName("p");
var num = pArray.length;
alert("<p>태그의 개수는 "+num);
}
</script>
</head>
<body>
<h3>자바스크립트란?
<button onclick="border()">border</button>
<button onclick="p()">p</button>
</h3>
<hr>
<p>자바스크립트는 웹 프로그래밍 언어로서 웹 페이지를 동적 변경시킬 수 있다.</p>
<p><span id="mySpan">C 언어</span>를 아는 사용자는 빠르게 학습할 수 있다.</p>
<p>이벤트 등 GUI 프로그래밍에 필요한 요소들이 있어 학습에 약간의 어려움이 있다.</p>
</body>
</html>
3.다음은 예제 5-7을 수정하여 출력한 결과이다. 예제에 버튼(show/hide)를 추가하고 자바스크립트코드를 삽입하여 버튼을 클릭하면 답이 보이게 하고, 다시 클릭하면 숨기도록 구현하였다. 웹 피이지를 작성하라.
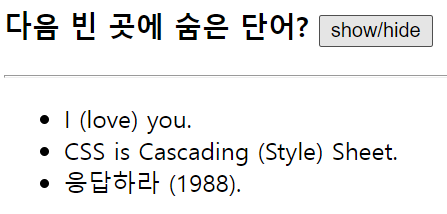
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>visibility 프로퍼티</title>
<style>
span{
visibility: hidden;
}
</style>
<script>
var flag=0;
function showhide() {
var spanArray = document.getElementsByTagName("span");
for(var i=0;i<spanArray.length;i++){
var span = spanArray[i];
if(flag==0){
span.style.visibility="visible";
}else if(flag==1){
span.style.visibility="hidden";
}
}
if(flag==0){
flag=1;
}else{
flag=0;
}
}
</script>
</head>
<body>
<h3>다음 빈 곳에 숨은 단어?
<button onclick="showhide()">show/hide</button></h3>
<hr>
<ul>
<li>I (<span>love</span>) you.</li>
<li>CSS is Cascading (<span>Style</span>) Sheet.</li>
<li>응답하라 (<span>1988</span>).</li>
</ul>
</body>
</html>
4.다음과 같이 계산식이 출력되고 '답'을 클릭하면 계산결과를 출력하는 웹 페이지를 작성하고자 한다. HTML 소스의 빈칸을 채우고 HTML페이지를 완성하라.
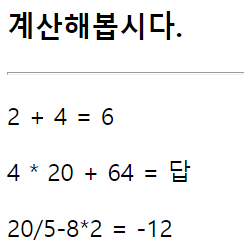
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>계산해봅시다.</title>
</head>
<body>
<h3>계산해봅시다.</h3>
<hr>
<p>2 + 4 = <span onclick="this.innerHTML=eval(2+4)">답</span></p>
<p>4 * 20 + 64 = <span onclick="this.innerHTML=eval(4*20+64)">답</span></p>
<p>20/5-8*2 = <span onclick="this.innerHTML=eval(20/5-8*2)">답</span></p>
</body>
</html>
5.다음 HTML 페이지를 출력하면 동일한 크기의 카드 4개가 겹쳐 있어, 맨 위에 배치된 카드만 보인다(예제 5-6참고). 이것은 각 카드 이미지의 z-index 스타일 프로퍼티 값을 다르게 설정하여 값이 클수록 위에 배치되도록 하였기 때문이다. 카드 이미지를 클릭하면 클릭된 카드가 맨 밑으로 들어가고 바로 밑의 카드가 나타나도록, 자바스크립트 코드를 작성하라.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>z-index 스타일 프로퍼티</title>
<style>
div{position: absolute;}
img{position: absolute;}
#
</style>
</head>
<body>
<h3>z-index 스타일 프로퍼티</h3>
<hr>
<div>
<img src="./media/spade-A.png" width="100" height="140" alt="스페이드A">
<img src="./media/spade-2.png" width="100" height="140" alt="스페이드2">
<img src="./media/spade-3.png" width="100" height="140" alt="스페이드3">
<img src="./media/spade-7.png" width="100" height="140" alt="스페이드4">
</div>
<script>
// z-index, left, top 모두 초기화
var imgArray = document.getElementsByTagName("img");
for(i=0; i<imgArray.length; i++) {
var obj = imgArray[i];
obj.style.zIndex=i;
obj.onclick = shuffle;
}
function shuffle() {
for(i=0; i<imgArray.length; i++) {
var obj = imgArray[i];
obj.style.zIndex++;
obj.style.zIndex %= imgArray.length;
}
}
</script>
</body>
</html>
6.DOM객체의 innerHTML 프로퍼티를 다루는 연습을 해보자. 다음과 같이 <p></p>태그로 출력된 텍스트에 마우스를 클릭하면, prompt() 창을 출력하고 사용자가 입력한 HTML 텍스트로 <p>태그의 내용을 바꾸는 웹 페이지를 작성하라.
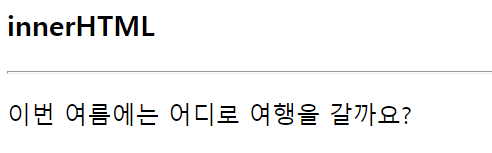
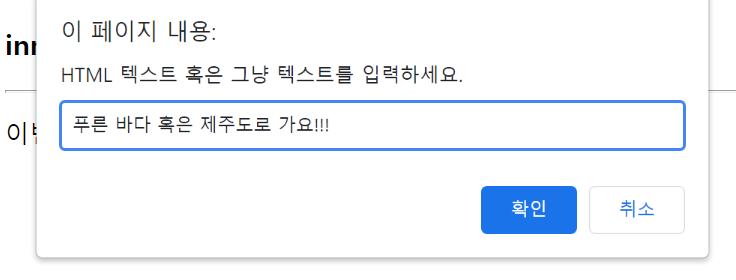
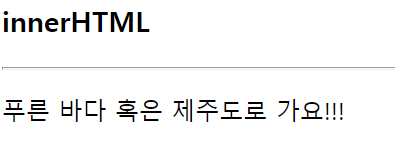
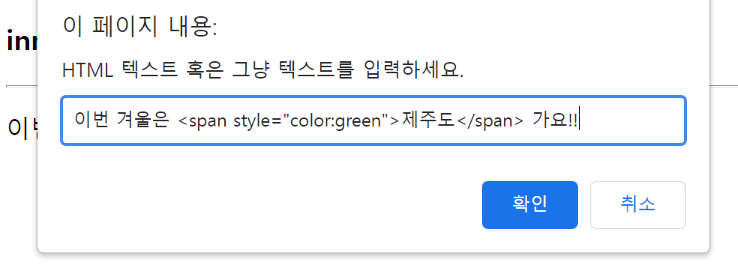
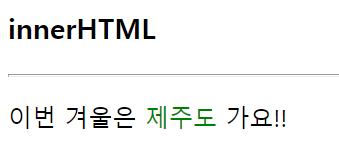
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>innerHTML</title>
<script>
function change(obj){
obj.innerHTML=prompt("HTML 텍스트 혹은 그냥 텍스트를 입력하세요.");
}
</script>
</head>
<body>
<h3>innerHTML</h3>
<hr>
<p onclick="change(this)">이번 여름에는 어디로 여행을 갈까요?</p>
</body>
</html>
7.이미지를 마우스로 클릭하면 이미지를 90도 회전시키는 웹 페이지를 작성하라.
(1)클릭하면 한 번만 90도 회전하기
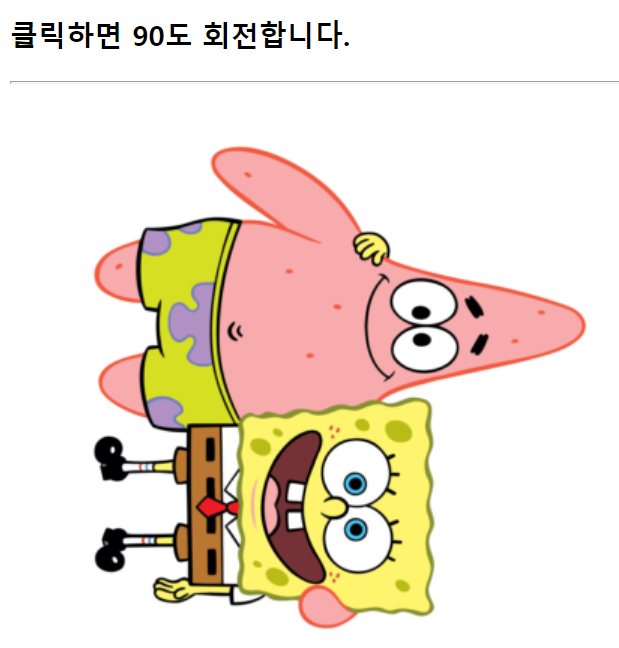
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>애니메이션</title>
<script>
function transform(obj){
obj.style.transform="rotate(90deg)"
}
</script>
</head>
<body>
<h3>클릭하면 90도 회전합니다.</h3>
<hr>
<img onclick="transform(this)" src="./media/spongebob.png">
</body>
</html>
(2)클릭할 때마다 90도씩 계속 회전하기
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>애니메이션</title>
<script>
var n=0;
function transform(obj){
n+=90;
obj.style.transform="rotate(" + n + "deg)"
}
</script>
</head>
<body>
<h3>클릭하면 90도 회전합니다.</h3>
<hr>
<img onclick="transform(this)" src="./media/spongebob.png">
</body>
</html>
8.이미지를 클릭할 때마다 옆으로 2,3,4배로 확대하여 출력하는 웹 페이지를 작성하라.
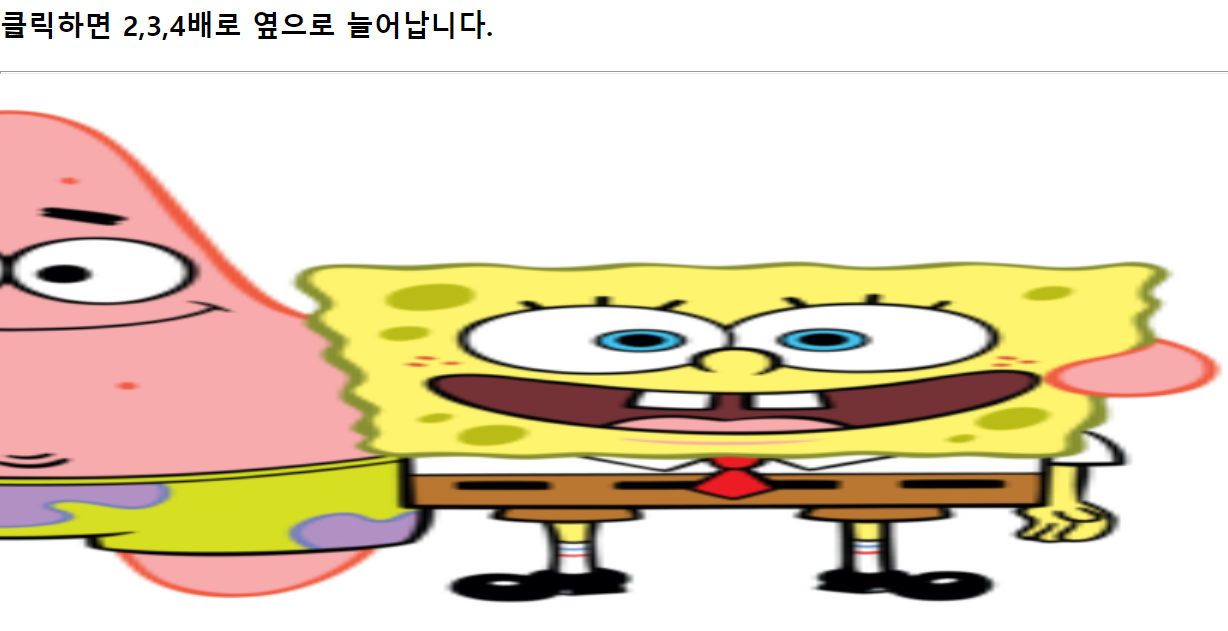
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>애니메이션</title>
<script>
var n=1;
function transform(obj){
if(n<4){
n+=1;
obj.style.transform="scale("+n+",1)";
}
}
</script>
</head>
<body>
<h3>클릭하면 2,3,4배로 옆으로 늘어납니다.</h3>
<hr>
<img onclick="transform(this)" src="./media/spongebob.png">
</body>
</html>
9.다음은 HTML페이지와 브라우저의 출력결과이다.
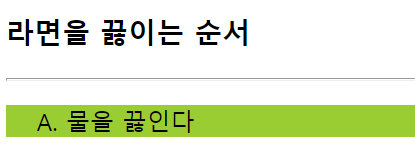
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>라면을 끓이는 순서</title>
</head>
<body>
<h3>라면을 끓이는 순서</h3>
<hr>
<ol type="A" style="background-color: yellowgreen;">
<li>물을 끓인다</li>
</ol>
</body>
</html>
yellowgreen 색으로 칠해진 영역은 <ol>이 출력도니 영역이다. 이 영역에 마우스로 클릭하면 다음과 같이 prompt() 함수를 출력하고, 사용자로부터 입력받은 문자열로 끓이는 순서를 하나씩 삽입하는 자바스크립트 코드를 작성하라.
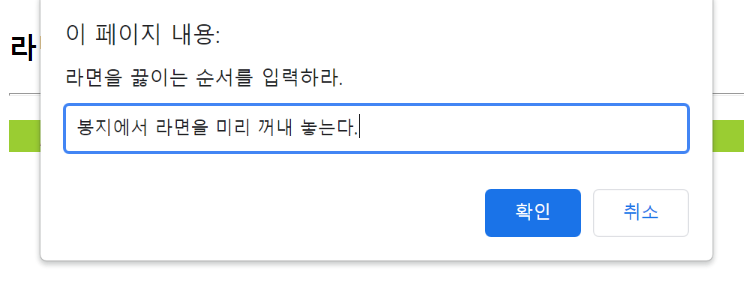
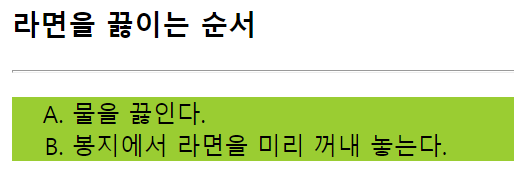
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>라면을 끓이는 순서</title>
<script>
function plus(){
var text=prompt("라면을 끓이는 순서를 입력하라.");
var ol = document.getElementsByTagName("ol")[0];
var newli = document.createElement("li");
newli.innerHTML = text;
ol.appendChild(newli); }
</script>
</head>
<body>
<h3>라면을 끓이는 순서</h3>
<hr>
<ol type="A" style="background-color: yellowgreen;" onclick="plus()">
<li>물을 끓인다.</li>
</ol>
</body>
</html>
10.다음 오른쪽 위 화면에서 답보기 버튼을 누르면 아래 화면과 같이 정답이 출력된다.
(1)정답은 다음 코드에서 <p> 객체를 동적으로 생성하여 삽입한다. 코드를 완성하라.
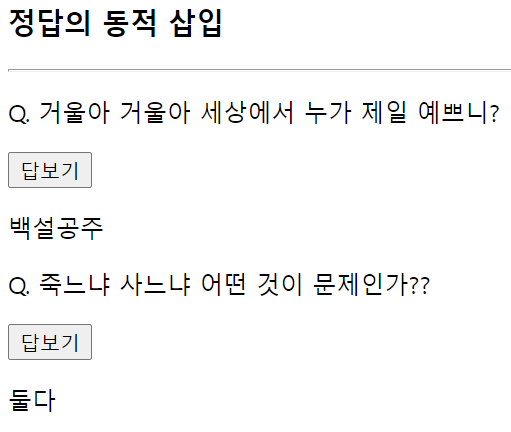
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>DOM 객체 동적 삽입</title>
<script>
function addAnswer(obj,text){
var newP = document.createElement("p");
newP.innerHTML = text;
obj.parentElement.appendChild(newP);
}
</script>
</head>
<body>
<h3>정답의 동적 삽입</h3>
<hr>
<div>
<p>Q. 거울아 거울아 세상에서 누가 제일 예쁘니?</p>
<button onclick="addAnswer(this,'백설공주')">답보기</button>
</div>
<div>
<p>Q. 죽느냐 사느냐 어떤 것이 문제인가??</p>
<button onclick="addAnswer(this,'둘다')">답보기</button>
</div>
</body>
</html>
(2)문제 (1)의 코드를 수정하여 정답으로 출력된 '백설 공주'나 '둘다'를 클릭하면 정답이 다시 제거되게 하라.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>DOM 객체 동적 삽입</title>
<script>
function addAnswer(obj,text){
var newP = document.createElement("p");
newP.innerHTML = text;
newP.addEventListener("click",
function(){
this.parentElement.removeChild(this);
});
obj.parentElement.appendChild(newP);
}
</script>
</head>
<body>
<h3>정답의 동적 삽입</h3>
<hr>
<div>
<p>Q. 거울아 거울아 세상에서 누가 제일 예쁘니?</p>
<button onclick="addAnswer(this,'백설공주')">답보기</button>
</div>
<div>
<p>Q. 죽느냐 사느냐 어떤 것이 문제인가??</p>
<button onclick="addAnswer(this,'둘다')">답보기</button>
</div>
</body>
</html>
'HTML+CSS+JavaScript' 카테고리의 다른 글
[HTML5+CSS3+Javascript 웹프로그래밍]10장 OpenChallenge (0) | 2021.11.21 |
---|---|
[HTML5+CSS3+Javascript 웹프로그래밍]9장 실습문제 (0) | 2021.11.14 |
[HTML5+CSS3+Javascript 웹프로그래밍]7장 실습문제 (0) | 2021.10.15 |
[HTML5+CSS3+Javascript 웹프로그래밍]7장 연습문제 (0) | 2021.10.15 |
[HTML5+CSS3+Javascript 웹프로그래밍]3장 실습문제 (0) | 2021.10.15 |